In this post, we’ll walk through the basics of WebAssembly and the possibilities that it brings from the perspective of a JavaScript developer. It is important to clarify that WebAssembly is a tool and not a technology: it augments rather than replaces JavaScript in web development.
What is WebAssembly?
WebAssembly, or WASM, is a binary code format close to assembly and independent of the language and the platform since WebAssembly can be compiled from other languages and can be executed on a browser (Web APIs) or a virtual machine. WebAssembly is an open standard whose main objective is to offer a closely native performance on the web while maintaining compatibility with the current ecosystems and standards.
Basic Concepts
Before we start digging into WebAssembly, we need to familiarize ourselves with some basic concepts around this code format to understand how it runs on the browser.
Modules
WebAssembly is organized into modules compiled in binary by the browser (or virtual machine). They can be seen the same way as ECMAScript modules, where modules contain functions and can import and export other functions.
Stack Machine
WebAssembly is a stack machine because it works under a system of instructions (ISA). These instructions allow the control of the loops, arithmetic operations, and memory access.
Getting started with WebAssembly
In order to facilitate everything as much as possible, we are going to use WebAssembly Studio. This tool is an online editor for WebAssembly that allows us to create WASM projects in a variety of languages (WAT, Rust, C, AssemblyScript, etc.).
Basic Example
For our example, we are going to use AssemblyScript, which looks familiar to TypeScript and compiles the binary using Binaryen with a small decrease in performance. AssemblyScript was primarily designed for JavaScript/TypeScript developers.

The multiply function receives two 32-bit integers and returns the result of their multiplication, all while exposing the function for use in our JavaScript code later.
Interface to use WebAssembly functions on JavaScript
WebAssembly was designed to be used with JavaScript and have an API that allows the integration of WASM modules with JavaScript.
So in order to be able to use the API, our first step is to load our WASM file as a resource. We can do this using a basic fetch.

Now we need to download the WASM file and compile it to get the WASM module. This step can be solved differently but we are going to use the instantiateStreaming method. Then we retrieve the instance from that module to be able to access the multiply function created on our WASM file.
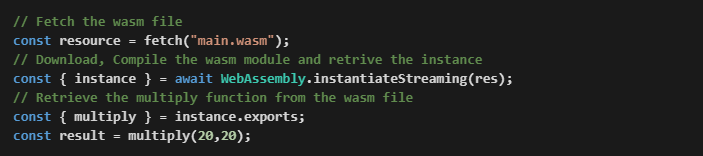
Comparison with Javascript
WebAssembly was made to improve the performance of our JavaScript applications since it is binary-based and uses the raw power of very performant languages. We are going to measure the time it takes to perform the multiply function for both implementations (WASM and JavaScript).
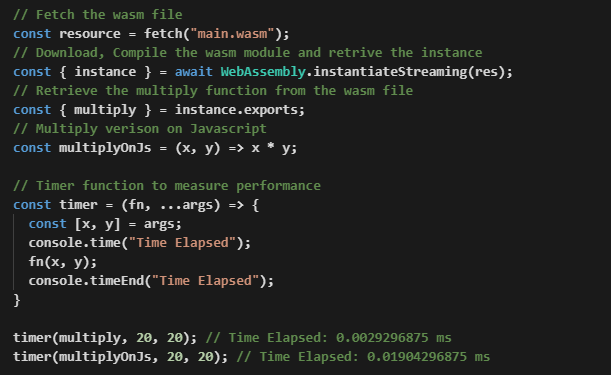
I made several executions of the code and the results vary on every run. But what we can see is how the WASM version is at least 60% faster than the pure JavaScript version.
Bonus Example
WebAssembly was made to improve performance on heavy tasks and one example of this can be image manipulation. So to test this, we’ll take the pixel manipulation with canvas example from MDN and add the same function to invert pixel colors but using WASM. This example is using the ImageData object to manipulate the pixels of a canvas.
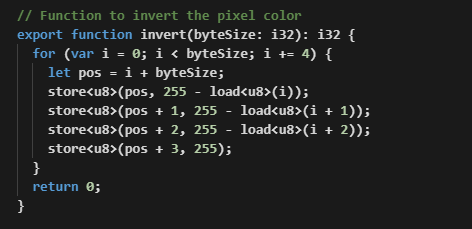
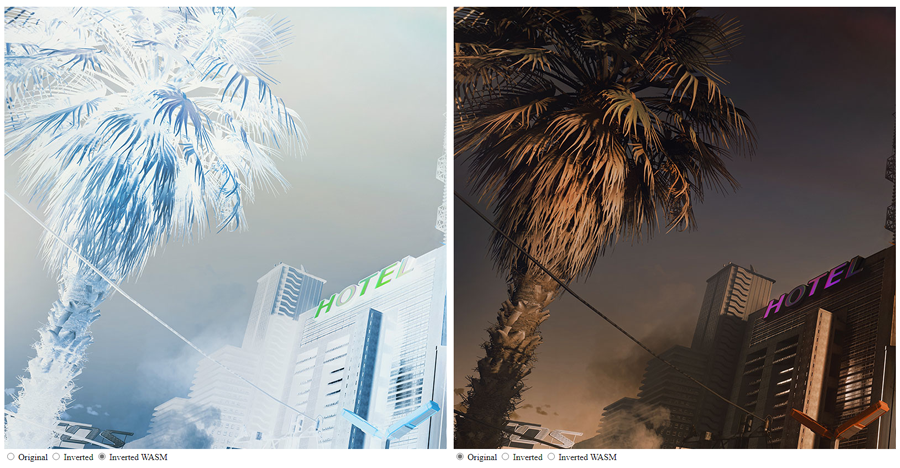
In this example, WebAssembly once again is faster, showing an improvement of 50 - 60% for average on each execution. This does not mean that everything we do in WebAssembly will always have these performance differences.
Bi-directional Communication between JavaScript and WebAssembly
In the previous examples we have accessed WASM functions from JavaScript, but WebAssembly allows bidirectional communication. What that means is that we can access the browser APIs. Let’s say we want to access the console from WASM. To accomplish this we can pass an object with import options when we are instantiating the WASM module.
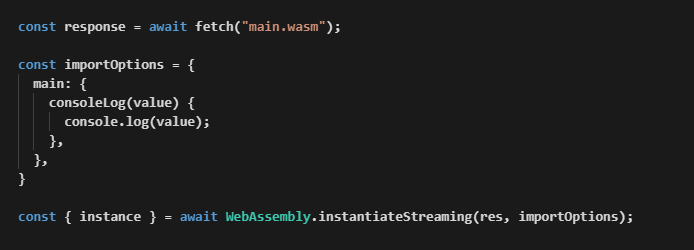
Then to use it on our AssemblyScript file, we should declare the function before we use it.

Conclusions
As you saw demonstrated above, WebAssembly allows bi-directional communication between JavaScript and WebAssembly modules to benefit from its high performance and easier access to the browser’s API. This does not mean that WebAssembly is going to replace JavaScript on applications that manage and consume APIs, but it does mean that it is a great tool to improve web performance.
WebAssembly is becoming more and more important for web development and is a great tool to improve applications for our clients. If you are interested in joining our team, please visit our careers page.